Do you need to add days to a date in JavaScript? Whether you are building a calendar app, a booking system, or a data analysis tool, you might encounter this problem at some point. However, working with dates and times in JavaScript can be tricky, especially when you need to perform calculations or comparisons. There are many factors to consider, such as leap years, daylight saving time, time zones, and formatting options.
In this blog, you will learn:
- What is the Date object?
- How to add days using three different methods
- Add days using third-party libraries
- There are a few real-time use cases, including counting days to date excluding weekends and holidays
- Using Temporal API to add days
- How to subtract days from a date in JavaScript.
The Date object in JavaScript
The JavaScript Date object provides a way to work with dates and times. With the Date
object, you can create, format, and manipulate dates. The following methods will help you add or subtract dates.
- setDate()
- getTime()
- Date.now()
Add days to date in JavaScript using the Date object
The most common and basic way to add days to a date in JavaScript is to use the Date object. The Date object represents a single moment in time and has various methods and properties that allow you to manipulate it.
To create a new Date object, you can use the following syntax:
let date = new Date(year, month, day, hour, minute, second, millisecond);
The parameters are optional. It can be omitted or specified in any order. If you omit some parameters, they will be set to their default values, such as the current date and time. If you specify invalid values, they will be adjusted accordingly.
Method 1: Using the setDate() and getDate() methods
The most common way of adding days to a date in JavaScript is using the setDate() and getDate() methods of the Date object. The setDate() method sets the day of the month for a given date object, and the getDate() method returns the day of the month for a given date object.
To add days to a date, you can use the following function:
// Get the current date
let today = new Date();
// Add 5 days to the current date
today.setDate(today.getDate() + 5);
console.log(today);
// Thu Jan 18 2024 19:38:25 GMT+0530 (India Standard Time)
As you can see, the new date is 5 days later than the current date. This method works for any number of days, positive or negative. If you want to subtract days from a date, just use a negative number.
For instance, it does not take into account leap years, daylight saving time, or time zones. It also modifies the original date object, which can cause unexpected side effects.
There are several methods for adding days to a date in JavaScript. Let’s take a look at how to add days to a date in JavaScript with three different approaches in this post.

To display the date in user locale-specific
format, you have to use The toLocalDateString() method.
Method 2: Add days to date using the Date constructor
Another way of adding days to a date in JavaScript is using the Date constructor. The Date constructor creates a new date object with a specified date and time. You can pass different arguments to the Date constructor, such as a string, a number, or multiple numbers.
Here’s an example to add 10 days:
let numberOfDaysToAdd = 10
let today = new Date();
let futureDate = new Date(today.getTime() + (numberOfDaysToAdd * 24 * 60 * 60 * 1000));
console.log(futureDate);
// Output: Fri Feb 10 2023 13:29:57 GMT+0530 (India Standard Time)
In this code, year, month, day, hours, minutes, seconds, and milliseconds are the parameters of the original date object. The numberOfDaysToAdd is a variable that represents the number of days you want to add to the date. The getTime() method returns the number of milliseconds since January 1st, 1970, for a given date object.
To add days to the date, you can multiply the number of days by the number of milliseconds in a day (24 hours * 60 minutes * 60 seconds * 1000 milliseconds), and add it to the original date’s milliseconds.
Method 3. Add days using the Date.now() method
You can use the Date.now()
method to add days to a date. The Date.now() method returns the current date and time as a number of milliseconds since January 1, 1970
. You can add the desired number of milliseconds
to this value to get a future date.
Here’s an example:
let numberOfDaysToAdd = 10
let futureDate = new Date(Date.now() + (numberOfDaysToAdd * 24 * 60 * 60 * 1000));
console.log(futureDate);
// Output: Fri Feb 10 2023 13:31:27 GMT+0530 (India Standard Time)
Using a library like Moment.js or Date-fns to add days
Moment.js
and Date-fns
offer a lot of features and functionality, such as parsing, validation, manipulation, comparison, display, and localization. These libraries also handle leap years, daylight saving time, and time zones correctly.Adding days to date using Date-fns
Moment.js
, you can use the add()
method, which takes a number and a unit of time as arguments.For example, to add 5 days to the current date using Moment.js
, you can do this:// Get the current date as a Moment object
let today = moment();
// Add 5 days to the current date
let newDate = today.add(5, 'days');
// Log the new date
console.log(newDate);
Adding days to date using Date-fns
Date-fns
, you can use the addDays()
function, which takes a date and a number of days as arguments.For example, to add 5 days to the current date using Date-fns
, you can do this:// Get the current date as a Date object
let today = new Date();
// Add 5 days to the current date
let newDate = dateFns.addDays(today, 5);
// Log the new date
console.log(newDate);
// Output: 2024-01-18T13:51:30.000Z
As you can see, both libraries produce similar results, but with different syntax and output formats. You can choose the one that suits your needs.
How to add days to a date JavaScript: Real-time use cases
Here are some code examples and use cases for adding days to a date in JavaScript:
1. Adding 7 days to the current date
Adding the number of days to the current date is a straightforward approach. For example, the following code adds 7 days
to the current date
.
let today = new Date();
let futureDate = new Date();
let numberOfDaysToAdd = 7
futureDate.setDate(today.getDate() + numberOfDaysToAdd);
console.log(futureDate);
// Output: Tue Feb 07 2023 13:32:59 GMT+0530 (India Standard Time)
With this approach, you can add one day to a date by changing the numberOfDaysToAdd
variable value to 1. To add 30 days
to the date, you can change the numberOfDaysToAdd
value to 30
.
2. Adding 14 days to a specific date string
Let’s assume you have a JavaScript date format YYYY-MM-DD string. You want to convert it to a date and add 14 days
it. You can use the following code:
let specificDate = new Date("2023-01-01");
const numberOfDaysToAdd = 14;
// 14 days x 24 hours x 60 minutes x 60 seconds x 1000 (1 millisecond) = 86400000 milliseconds
const millisecondsPerDay = 86400000;
let futureDate = new Date(specificDate.getTime() + (numberOfDaysToAdd * millisecondsPerDay));
console.log(futureDate);
// Output: Sun Jan 15 2023 05:30:00 GMT+0530 (India Standard Time)
"2023-01-01"
, considering milliseconds in a day.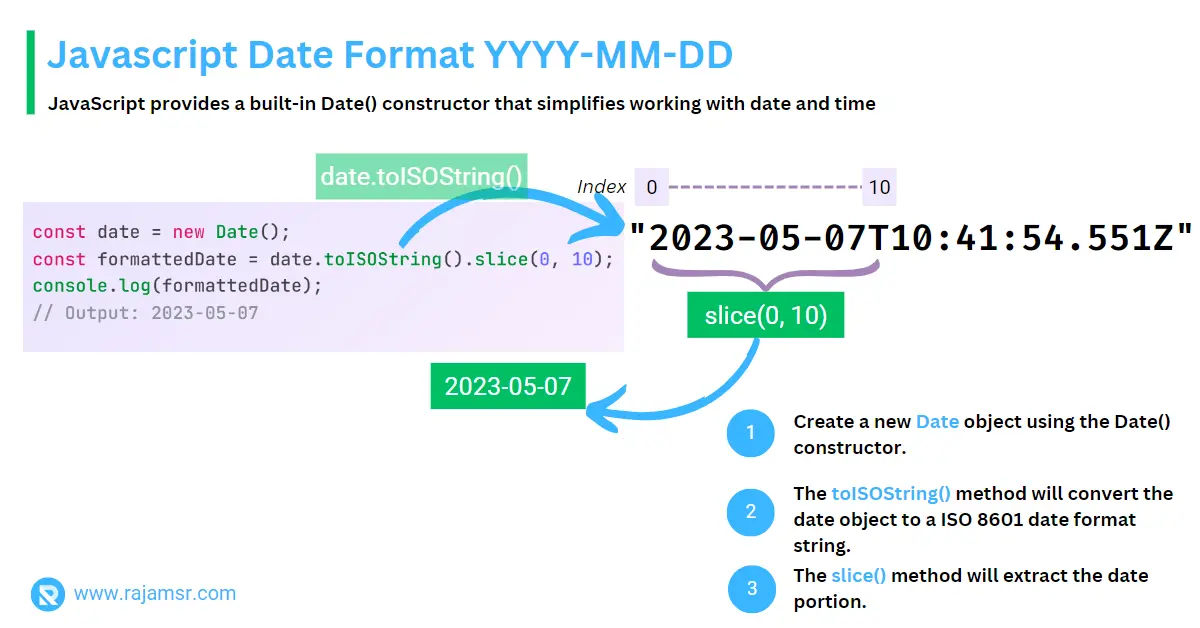
3. Add days to date excluding weekends and holidays
Here’s an example code to add a specified number of business days (excluding weekends
and holidays
) to a given date in JavaScript:
function addBusinessDays(startDate, daysToAdd) {
const millisecondsPerDay = 86400000;
let count = 0;
while (count < daysToAdd) {
startDate.setTime(startDate.getTime() + millisecondsPerDay);
let day = startDate.getDay();
let weekends = [0, 6]; // 0 - Sunday, 6 - Saturday
if (!weekends.includes(day)) {
// Check if the date is not a weekend
const dateString = startDate.toISOString().slice(0, 10);
if (!isHoliday(dateString)) {
// if day is not weekend and holiday, count it as business day.
count++;
}
}
}
return startDate;
}
function isHoliday(dateString) {
// Add all your holiday dates as strings in an array
const holidays = ['2023-01-26', '2023-02-05'];
return holidays.includes(dateString);
}
const startDate = new Date('2023-01-01');
const daysToAdd = 60;
const businessDate = addBusinessDays(startDate, daysToAdd);
console.log(businessDate.toISOString().slice(0, 10));
// Output: 2023-04-03
In this example, the addBusinessDays()
function takes a startDate
and daysToAdd
as inputs and returns a new date that is daysToAdd
business days ahead of the startDate
. The isHoliday()
function checks if a given date is a holiday by comparing it to an array of holiday dates.
Using the toISOString() method, the date is converted to an ISO format (yyyy-mm-dd) string.
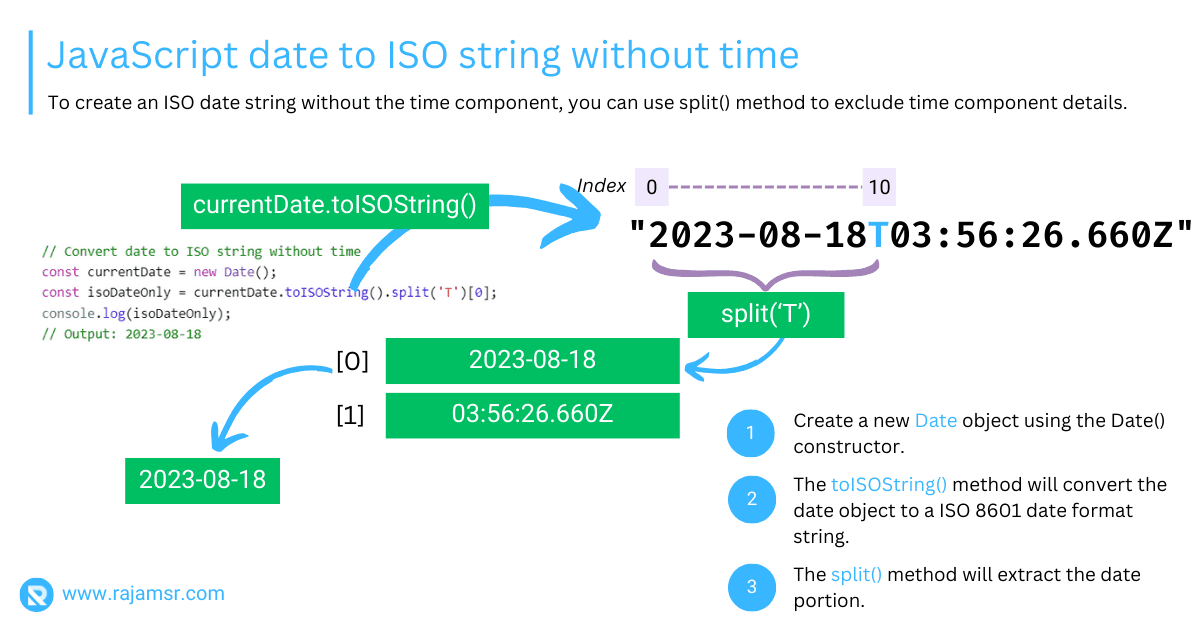
How to add days to a Date in JavaScript using the Temporal API
The Temporal API is a new and modern way to work with dates and times in JavaScript. It is currently a proposal and not yet part of the official JavaScript specification, but you can use it with a polyfill or a transpiler. The Temporal API provides various classes and methods that make date and time manipulation easier and more consistent.
To create a new Temporal object, you can use the following syntax:
let date = Temporal.PlainDate.from(year, month, day);
The parameters are required and must be valid values. If you pass invalid values, an error will be thrown. For example:
// Creates a PlainDate object with January 1, 2023
let date1 = Temporal.PlainDate.from(2023, 1, 1);
// Throws an error: RangeError: Invalid month 13
let date2 = Temporal.PlainDate.from(2023, 13, 1);
To add days to a date in JavaScript using the Temporal API, you can use the add (duration)
method. Which returns a new Temporal object that is the result of adding a duration to the original Temporal object. The duration is an object that specifies the amount and unit of time to add. For example, {days: 10}
means 10 days.
Here is an example of how to use this method to add days to a date in JavaScript:
// Creates a PlainDate object with January 1, 2023
let date = Temporal.PlainDate.from(2023, 1, 1);
console.log(date);
// Output: "2023-01-01"
// The number of days to add to the date
let daysToAdd = 10;
// Creates a duration object with 10 days
let duration = {days: daysToAdd};
date = date.add(duration);
console.log(date);
// Output: "2023-01-11"
How to format Dates in JavaScript
After adding days to a date in JavaScript, you might want to format the date in a human-readable way. For example, you might want to display the date as "January 11, 2023"
or "11/01/2023"
or "Wednesday, 11th of January, 2023"
.
To format dates in JavaScript, you can use the JavaScript toLocaleDateString (locales, options) method. It returns a string with a language-sensitive representation of the date. The locales parameter is an array of strings that specify the language and region to use for formatting.
The options parameter is an object that specifies the format options, such as the date style, the time style, the calendar, the numbering system, etc. Here is an example of how to format a date:
// Creates a Date object with January 11, 2023, 00:00:00
let date = new Date(2023, 0, 11);
console.log(date.toLocaleDateString()); // "1/11/2023"
console.log(date.toLocaleDateString("en-US")); // "1/11/2023"
console.log(date.toLocaleDateString("en-GB")); // "11/01/2023"
console.log(date.toLocaleDateString("fr-FR")); // "11/01/2023"
console.log(date.toLocaleDateString("en-US", {dateStyle: "short"})); // "1/11/23"
console.log(date.toLocaleDateString("en-US", {dateStyle: "full"}));
// "Wednesday, January 11, 2023"
JavaScript toDateString() returns a string with a default representation of the date. The format is not guaranteed to be consistent across different browsers and platforms. For example:
// Creates a Date object with January 11, 2023, 00:00:00
let date = new Date(2023, 0, 11);
console.log(date.toDateString());
// "Wed Jan 11 2023"
How to compare Dates in JavaScript
Another common task that involves adding days to a date in JavaScript is comparing dates. For example, you might want to check if a date is before, after, or equal to another date, or if a date is within a certain range of dates.
To compare dates in JavaScript, you can use the following methods:
// Creates a Date object with January 1, 2023, 00:00:00
let date1 = new Date(2023, 0, 1);
// Creates a Date object with January 11, 2023, 00:00:00
let date2 = new Date(2023, 0, 11);
console.log(date1.getTime()); // Output: "1672531200000"
console.log(date2.getTime()); // Output: "1673344800000"
console.log(date1.getTime() < date2.getTime());
// Output: "true" (date1 is before date2)
console.log(date1.getTime() > date2.getTime());
// Output: "false" (date1 is not after date2)
console.log(date1.getTime() === date2.getTime());
// Output: "false" (date1 is not equal to date2)
How to handle time zones in JavaScript
One of the challenges of working with dates and times in JavaScript is handling time zones. Time zones are regions of the earth that have a standard time, which is usually offset from the Coordinated Universal Time (UTC) by a certain number of hours.
For example, India Standard Time (IST)
is UTC+05:30, which means it is 5 hours and 30 minutes
ahead of UTC.
Time zones can affect how you add days to a date in JavaScript, as different time zones may have different dates and times for the same moment. For example, if you add one day to January 1, 2023, 00:00:00 in IST
, you will get January 2, 2023, 00:00:00
in IST. However, if you add one day to January 1, 2023, 00:00:00
in UTC, you will get January 1, 2023, 19:00:00
in IST, which is still the same day.
To handle time zones in JavaScript, you can use the following methods:
getTimezoneOffset()
: Returns the difference in minutes between the local time zone and UTC of the Date object.toUTCString()
: Returns a string with a UTC representation of the date.toLocaleString (locales, options)
: Returns a string with a language-sensitive representation of the date in a specific time zone. The locales parameter is an array of strings that specify the language and region to use for formatting. The options parameter is an object that specifies the format options, such as the date style, the time style, the time zone, the calendar, the numbering system, etc.
// Creates a Date object with January 1, 2024, 00:00:00 in the local time zone
let date = new Date(2024, 0, 1);
console.log(date.getTimezoneOffset());
// Output: "-330" (the local time zone is IST, which is UTC+05:30)
console.log(date.toUTCString());
// Output "Sun, 31 Dec 2023 18:30:00 GMT" (the UTC equivalent of the date)
console.log(date.toLocaleString());
// Output "1/1/2024, 12:00:00 AM" (default format in the local time zone)
Subtract days from date
JavaScript provides multiple ways to subtract days from a date. You can create a new Date
object, get the date value, and then subtract the number of days you want to subtract.
For example, date subtraction
in JavaScript:
// Example 1: Subtract 7 days from current date
let date = new Date();
let numberOfDaysToSubtract = 7;
date.setDate(date.getDate() - numberOfDaysToSubtract);
console.log(date)
// Output: Tue Jan 24 2023 13:43:19 GMT+0530 (India Standard Time)
// Example 2: Subtract 15 days from specific date
let specificDate = new Date("2023-01-01");
let numberOfDaysToSubtract = 15;
let futureDate = new Date(specificDate.getTime() - (numberOfDaysToSubtract * 24 * 60 * 60 * 1000));
console.log(futureDate);
// Output: Sat Dec 17 2022 05:30:00 GMT+0530 (India Standard Time)
Conclusion
In conclusion, manipulating dates is a common requirement in web development and an important skill for any web developer to have.
We looked at three different JavaScript methods for adding days to date:
- Add the number of days to the current date using the
setDate()
method. - Add the number of
milliseconds since January 1, 1970
, to the current date using thegetTime()
method - Add the number of milliseconds to the current date using the
Date.now()
method.
In addition to the above methods, we looked into how to add the number of days to a date string and how to add days excluding weekends
and holidays
. Using setDate()
and the Date()
object, you can subtract the number of days from a date.
Adding days to date is an essential part of web development, whether it’s for scheduling appointments, calculating deadlines, or tracking events. By becoming familiar with the different methods for adding days to a date in JavaScript, web developers can increase their productivity and create better web applications.