Are you looking for a JavaScript startsWith() alternative that can handle more complex scenarios? If you are working with strings in JavaScript, you might have encountered situations where you need to check if a string starts with a certain pattern, such as a prefix, a substring, or a regular expression. The built-in startsWith()
method can only check for exact matches of prefixes, which can be limiting in some cases.
In this blog post, I will show you:
- The syntax and parameters of the method
- How to check if a string starts with the substring
- JavaScript
startsWith()
alternative methods startsWith()
method with case-insensitive- How to implement startsWith() in old browsers
So let’s dive in and discover the power of the startsWith()
method!
JavaScript string startsWith() method
JavaScript string startsWith()
method determines whether a string starts with a specific string and returns true if the string begins with characters from another string; otherwise, it returns false. The JavaScript startsWith()
method is case-sensitive.
The syntax for the startsWith()
string method is:
string.startsWith(searchString, position);
Parameter | Description |
---|---|
string | The input string to be searched. |
searchString | The character or string to search for. |
position | Position optional. Represents where the search should start. If the position is ignored, it will search from the position 0. |
How to use startsWith() JavaScript method?
startsWith()
JavaScript string method, you simply call the method on a string and pass in the searchString
parameter.If you want to use the startsWith()
method from a specific index, you can pass in the position parameter. This is really useful when doing an incremental search on a string.
Here’s an example:
let inputString = "Hello JavaScript";
let startsWithHello = inputString.startsWith("Hello");
console.log(startsWithHello);
// Output: true
In the above example, we’re checking whether the JavaScript text begins with a "Hello"
string. In this example, the input string "Hello JavaScript"
starts with "Hello"
, so startsWith()
the method returns true
.
let inputString = "Hello JavaScript";
let startsWithJs = inputString.startsWith("JavaScript", 6);
console.log(startsWithJs);
// Output: true
In the startsWith()
method, we can also specify the start position. In this example, we’re starting the search at position 6, which means we’re checking if "JavaScript"
is at position 6 or later in the string.
There is an identical opposite method to the startsWith()
method. To check whether a string ends with a specific substring or character, you can use the JavaScript endsWith() method.
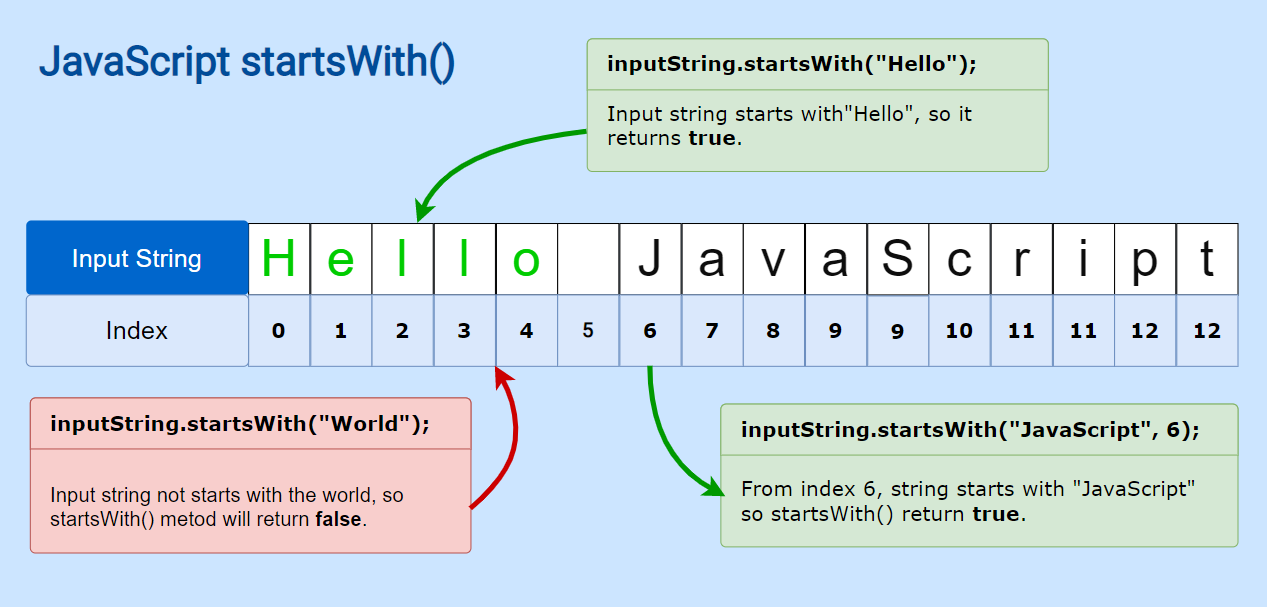
JavaScript startsWith() alternative methods
What are the JavaScript startsWith()
alternative methods? To check if a string begins with a substring, you can use different methods in JavaScript besides startsWith(). For example, indexOf() and lastIndexOf() methods return 0 when they find the substring at the start of the string. Similarly, slice() and substring() methods return true when the part they extract matches the substring.
Here we will see five different JavaScript startsWith()
alternative methods:
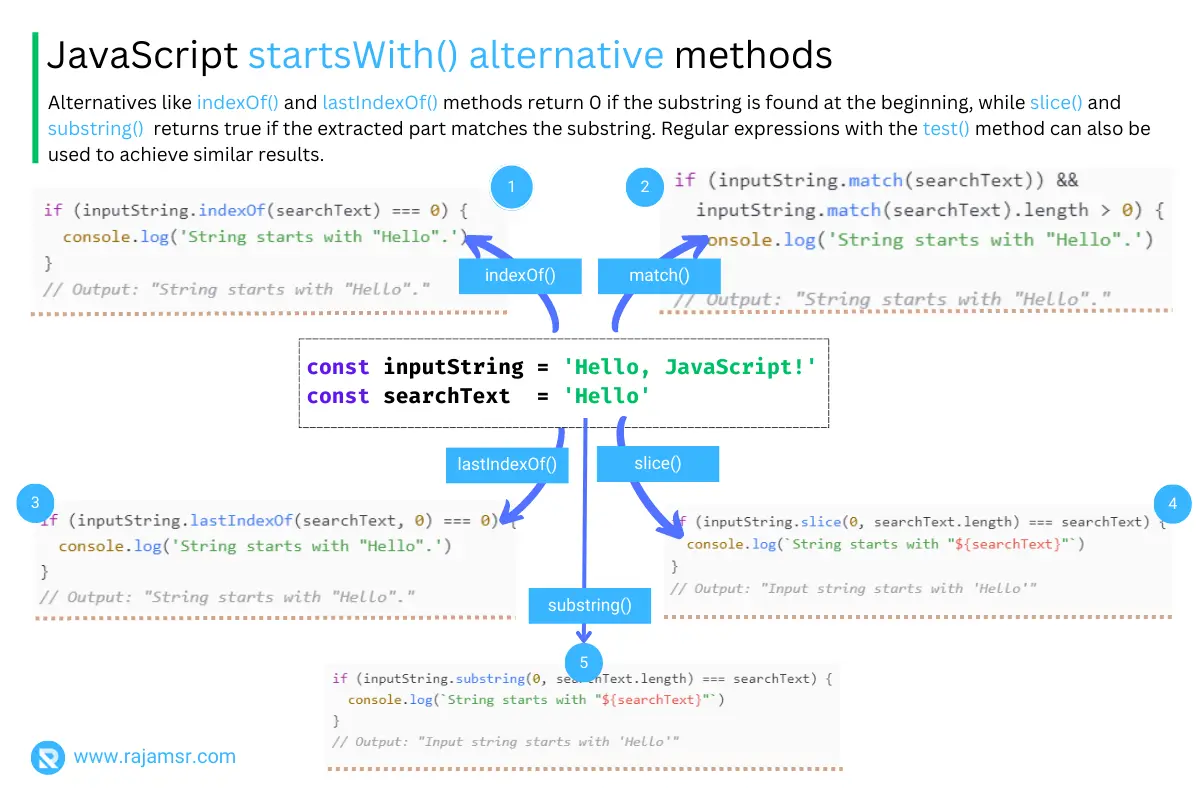
1. JavaScript startsWith() alternative using indexOf() method
JavaScript indexOf() method returns the position of the first occurrence of a specified value in a string. The indexOf()
method returns -1 when the value you are searching for is not in the string. The string or character you are searching for is at the beginning of the string when the indexOf()
method returns 0.
You can use the indexOf()
method as one of the startsWith()
alternative methods.
const inputString = 'Hello, JavaScript!'
const searchText = 'Hello'
if (inputString.indexOf(searchText) === 0) {
console.log('String starts with "Hello".')
}
// Output: "String starts with "Hello"."
2. Using lastIndexOf() as alternative method
The JavaScript lastIndexOf()
method returns the last occurrence of a substring in the string. If the lastIndexOf()
method returns 0, then you can confirm the string starts with the specified substring. Set the start index to 0 to begin from the string’s first character.
const inputString = 'Hello, JavaScript!'
const searchText = 'Hello'
if (inputString.lastIndexOf(searchText, 0) === 0) {
console.log('String starts with "Hello".')
}
// Output: "String starts with "Hello"."
3. Using match() method as JavaScript startsWith() alternative
This method searches a string for a specified value and returns that value as a string. It returns null when it does not find the value.
const inputString = 'Hello, JavaScript!'
const searchText = 'Hello'
if (inputString.match(searchText) && inputString.match(searchText).length > 0) {
console.log('String starts with "Hello".')
}
// Output: "String starts with "Hello"."
4. Using JavaScript slice() method as startsWith() alternative
The JavaScript slice() method extracts a portion of a string and returns a new string. It takes two parameters: the starting index
and the ending index
.
const inputString = 'Hello, JavaScript!'
const searchText = 'Hello'
if (inputString.slice(0, searchText.length) === searchText) {
console.log(`String starts with "${searchText}"`)
}
// Output: "Input string starts with 'Hello'"
5. The substring() as alternative to startsWith() method
The JavaScript substring() method extracts a portion of a string and returns it as a new string. It takes two parameters: the starting index (inclusive)
and the ending index (exclusive)
of the desired substring.
const inputString = 'Hello, JavaScript!'
const searchText = 'Hello'
if (inputString.substring(0, searchText.length) === searchText) {
console.log(`String starts with "${searchText}"`)
}
// Output: "Input string starts with 'Hello'"
You can use these startsWith()
alternative methods to accomplish the same result as the startsWith()
method.
JavaScript startsWith() alternative methods performance
The startsWith()
method is the most efficient and readable way to check if a string begins with a certain value. Other methods, such as slicing
, regex
, or indexOf()
, perform worse than startsWith()
in terms of performance and clarity.
I measured that startsWith() can handle 157,687
operations per second, while the other methods lag behind.
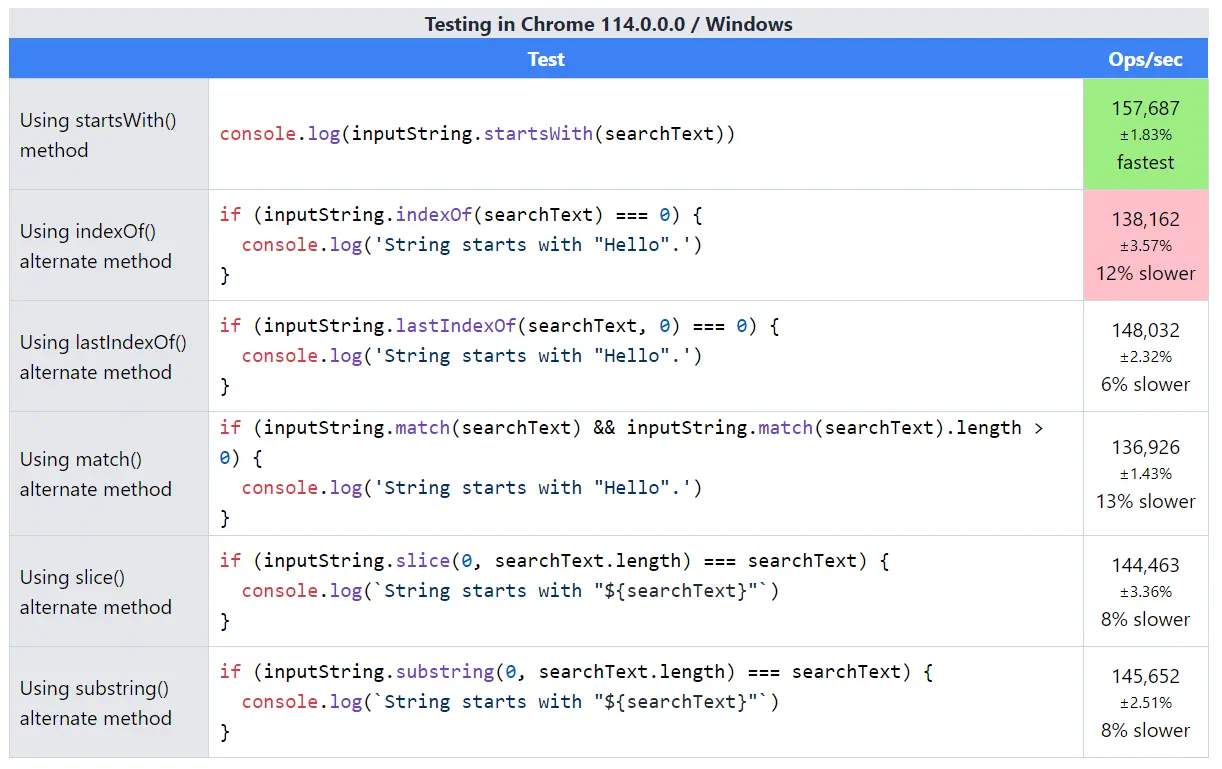
Using JavaScript string startsWith() method in real-world scenarios
The following are a few real-world instances of how to use the startsWith()
string method:
1. Validating phone number string starts with country code 1
When accepting user input, it’s often necessary to check if a JavaScript string starts with a certain character or substring. For example, let’s say you’re building a form where users can input their phone numbers, but you want to make sure they start with the country code 1.
You can use the string startsWith()
method to check this:
let phoneNumber = "1-999-999-9999";
if (phoneNumber.startsWith("1-")) {
console.log("Valid phone number");
} else {
console.log("Invalid phone number");
}
// Ouput: "Valid phone number"
2. Checking for URL protocol using string startswith() JavaScript method
When working with URLs
, it’s important to check if they start with a certain protocol, such as "http://"
or "https://"
. You can use the JavaScript string startsWith()
method to check for this:
// Check whether the URL is secure, insecure or Invalid
let url = "https://www.rajamsr.com";
if (url.startsWith("http://")) {
console.log(`Insecure URL ${url}`);
} else if (url.startsWith("https://")) {
console.log(`Secure URL ${url}`);
} else {
console.log(`Invalid URL ${url}`);
}
// Output: "Secure URL https://www.rajamsr.com"
3. Filtering array elements using startswith() in JavaScript
This is a typical scenario: from an array, you want to filter out elements based on whether they start with a specific character or string.
In the following example, to check if a string starts with "J"
we can use the JavaScript array filter()
method along with the startsWith()
method:
let months = ["Jan", "Feb", "Mar", "Apr", "May", "Jun"];
let filteredMonths = months.filter(m => m.startsWith("J"));
console.log(filteredMonths);
// Output: ["Jan", "Jun"]
4. Formatting URL using startsWith() JavaScript string method
To request a URL with an HTTP client, you must format the URL properly. In the following code example, the startsWith()
method is used to check whether the URL begins with https. If not, then add the https://
protocol before the URL.
let urls = ['https://www.google.com', 'www.bing.com'];
let formattedUrls = urls.map(url => {
return (url.startsWith('https://')) ? url : `https://${url}`;
});
console.log(formattedUrls);
// Output: ["https://www.google.com", "https://www.bing.com"]
5. Formatting file path
The JavaScript string format allows for precise control over the formatting of text within a string. In some cases, you may want to combine the base directory and path to build the complete path. Without the startsWith()
function, the below code will result in a double back slash or no path separator in some cases. This check is especially important when we are getting input from other methods or from users.
You can use the string startsWith()
method in JavaScript to achieve this:
let base = "C:\\Softwares";
let dir = 'JavaScript'
if (dir.startsWith("\\")) {
console.log(`${base}${dir}`);
} else {
console.log(`${base}\\${dir}`);
}
// Output: "C:\Softwares\JavaScript"
These are a few examples of how you can use the JavaScript string startsWith()
method in real-time use cases.
Check JavaScript string begins with using startsWith() multiple values
Sometimes to check string start with specific substring you have to use the startsWith()
method with multiple values. For example, to ensure the URL starts with either http
or https
, you can combine two startsWith()
methods with logical or (||) operators.
let url = "https://www.rajamsr.com";
if (url.startsWith("http://") || url.startsWith("https://")) {
console.log(`Valid URL ${url}`);
}
else {
console.log('Invalid URL format.')
}
// Output: "Valid URL https://www.rajamsr.com"
JavaScript startswith() case insensitive
The startsWith()
method is case-sensitive by default. In some cases, you have to check whether the string starts with a specific substring, regardless of the case.
In this instance, you can convert both the input and search string to JavaScript Lowercase or JavaScript Uppercase before calling the startsWith()
method to ensure that it works as expected regardless of the case.
Let’s see an example of how to startswith() case insensitive:
const startsWithIgnoreCase = (inputString, searchString) =>
{
const loweredInputString = inputString.toLocaleLowerCase();
return loweredInputString.startsWith(searchString.toLocaleLowerCase())
}
console.log(startsWithIgnoreCase('Hello, JavaScript', 'Hello'));
// Output: true
console.log(startsWithIgnoreCase('HELLO, JAVASCRIPT', 'hello'));
// Output: true
startsWithIgnoreCase()
checks if a string begins with another string. It doesn’t care about uppercase or lowercase letters. It makes both strings lowercase first to make it case insensitive. Then it uses a built-in method to compare them.How to implement startsWith() in older browsers
The startsWith()
method, which was introduced in ECMAScript 6, is enabled by modern browsers. However, if you need to support older browsers, this approach may cause problems. You can use a polyfill function to provide startsWith()
support for those browsers in that situation.
startsWith()
polyfill function:if (!String.prototype.startsWith) {
String.prototype.startsWith = function(searchString, position) {
position = position || 0;
return this.substr(position, searchString.length) === searchString;
};
}
Conclusion
The JavaScript startsWith()
method is an important method for JavaScript developers who need to verify and manipulate strings in their code. With its straightforward syntax and multiple use cases, it provides a convenient and efficient way to perform string comparisons and extractions.
- You can use the
startsWith()
method to validate user input, verify URL protocol, filter array elements that starts with a specific substring, and format URL or file names. - The
startsWith()
in JavaScript is case-sensitive by default. You must take extra steps to work in case insensitive mode, either by using stringlowercase
oruppercase
methods. - To accomplish the same functionality, you can use
startsWith()
alternative methods such asindexOf()
,match()
, orslice()
. - The
startsWith()
function in JavaScript does not support regular expressions. - Use the polyfill to enable the
startsWith()
method if your user is using an older browser, such as Internet Explorer 11 or earlier.
Whether you’re checking for file extensions, verifying user input, or extracting keywords, the startsWith()
method is a must-know method in JavaScript.