Are you tired of sifting through endless lines of code to extract the precise data you need in JavaScript? Look no further, because JavaScript slice()
is here to revolutionize your string manipulation game.
The JavaScript slice()
string method allows you to extract a portion of a string or an array and return it as a new string or array. The slice method does not modify the original string or array but instead creates a new one.
In this blog, we will explore:
- What is a
slice()
in JavaScript? - How do I use it?
- How it will work with strings, arrays, and objects
- Various use cases of the
slice()
method - Common pitfalls to watch out for when using the slice method
Let’s dive deep.
What is JavaScript slice() string method?
The slice()
method is one of the JavaScript String methods used to extract a portion of a string or an array and return it as a new string or array. The string slice()
method takes two parameters, startIndex
and endIndex
. The start and end index parameters are optional, but it is recommended to use them for more accurate results.
The syntax for the slice()
method is as follows:
string.slice(startIndex, endIndex);
array.slice(startIndex, endIndex);
Parameter | Description |
---|---|
startIndex | Optional. The start parameter is the index of the string or array where you want to start extracting the portion. |
endIndex | Optional. The end parameter is the index of the string or array where you want to stop extracting the portion. |
JavaScript slice() for Strings
How to slice a string in JavaScript?
Let’s start with how to slice a string in JavaScript. In JavaScript, a string is a sequence of characters enclosed in quotes. The slice()
method allows us to extract portions of a string based on specific indexes.
Imagine you have a long string, and you only want to extract a specific portion from it. By using positive indexes, you can easily extract substrings.
const message = "Hello, World!";
const substring = message.slice(7);
console.log(substring);
// Output: "World!"
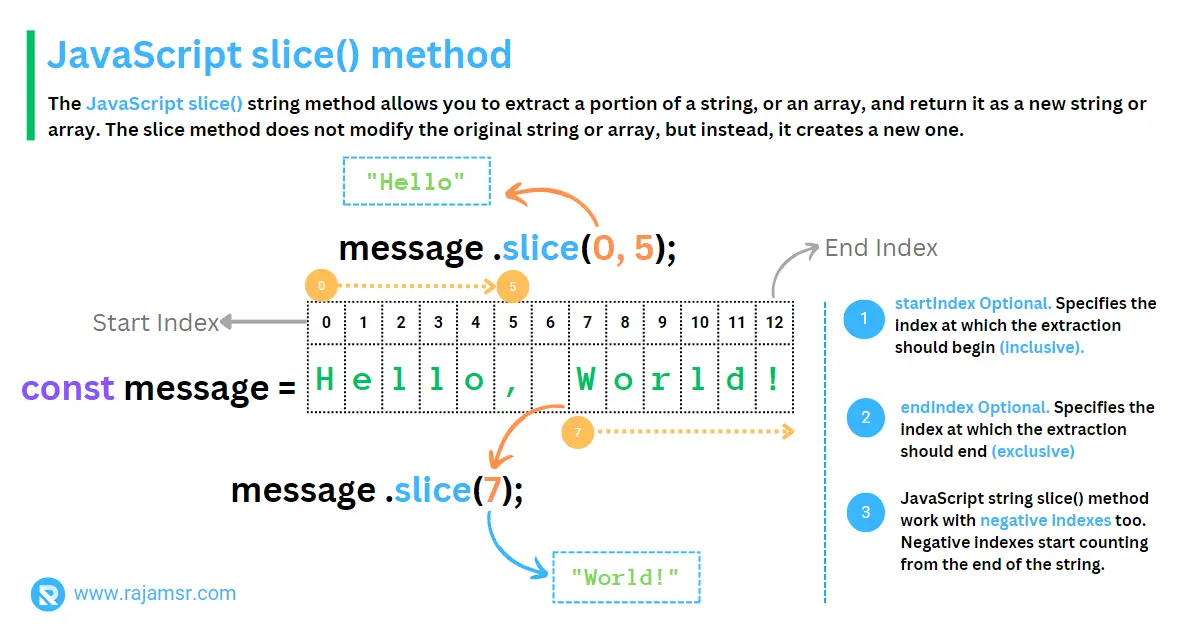
JavaScript slice() string by character
Sometimes, you may need to extract substrings based on specific characters rather than indexes. You can combine the JavaScript indexOf() method with a slice()
to achieve this.
const message = "Hello, JavaScript!";
const character = "J";
const substring = message.slice(message.indexOf(character));
console.log(substring);
// Output: "JavaScript!"
With this approach, you can slice string at character.
Working with slice() negative index
The JavaScript string slice()
method isn’t limited to positive indexes. It can work its magic with negative indexes too! Negative indexes start counting from the end of the string.
Let’s see it in action:
const message = "Hello, JavaScript!";
const substring = message.slice(-11);
console.log(substring);
// Output: "JavaScript!"
JavaScript slice() for Arrays
slice()
method opens up a world of possibilities for manipulating them. It allows us to extract specific elements and create new arrays effortlessly.Extracting array elements
Extracting specific elements from an array is straightforward with the JavaScript slice()
method. Simply provide the start and end indexes!
const numbers = [1, 2, 3, 4, 5];
const slicedNumbers = numbers.slice(3, 5);
console.log(slicedNumbers)
// Output: [4, 5]
JavaScript Slice() vs. Splice()
Slice()
and splice() might sound similar, but they have distinct purposes. While slice()
extracting elements from an array, splice()
modifies the array itself. It’s essential to understand the differences between the two to avoid confusion.
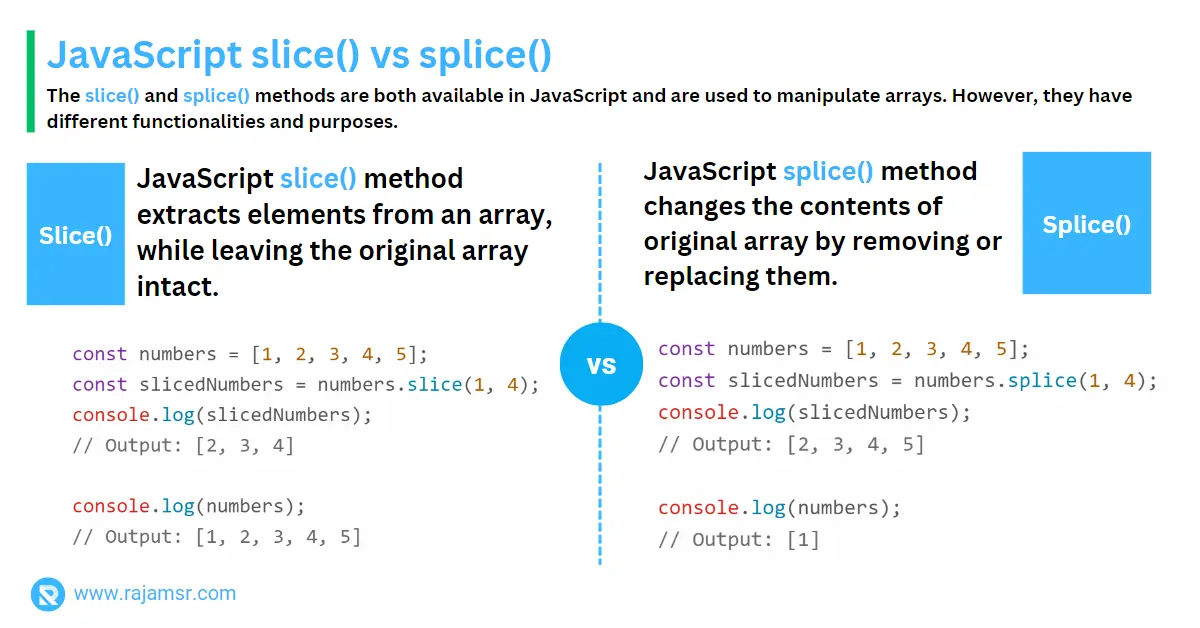
Slice last element of an array
Extracting the last element of an array is a common task, and JavaScript slice makes it a breeze. Just use a negative index to specify the position!
const numbers = [1, 2, 3, 4, 5];
const lastElement = numbers.slice(-1);
console.log(lastElement);
// Output: [5]
Alternatively, you can use the JavaScript array length property to get the number of elements in an array. If -1
is deducted from the length, then it will return the last element from an array.
const numbers = [1, 2, 3, 4, 5];
const lastElement = numbers.slice(numbers.length - 1);
console.log(lastElement);
// Output: [5]
JavaScript slice() for Objects
While arrays and strings dominate JavaScript slice()
discussions, we can’t overlook their potential with object properties. Object properties are accessed differently, but slice()
can still come to the rescue.
Extracting object properties
To extract object properties, we can utilize the JavaScript Object.keys() method in combination with the JavaScript slice()
. Let’s see it in action:
const person =
{
name: "John",
age: 25,
city: "New York"
};
const keys = Object.keys(person);
const slicedKeys = keys.slice(1);
console.log(slicedKeys);
// Output: ["age", "city"]
Usages of the slice() method in JavaScript
1. Extracting a portion of an array
The slice()
method can be used to extract a portion of an array. For example, you can use the slice method to extract the first half of an array or to extract a portion of an array based on specific criteria.
For example, let’s say we have an array of numbers and we want to create a new array with the first three numbers:
const numbers = [1, 2, 3, 4, 5];
const slicedNumbers = numbers.slice(0, 3);
console.log(slicedNumbers);
// Output: [1, 2, 3]
2. Copying an array
The slice()
method can also be used to create a shallow copy of an array.
Let’s say we have a number array from 1 to 5
. If you want to create a copy of this array, you can use the following method:
const numbers = [1, 2, 3, 4, 5];
const copiedNumbers = numbers.slice();
console.log(copiedNumbers)
// Output: [1, 2, 3, 4, 5]
If you are making changes to it copiedArray
, it will not impact the original array numbers.
3. Removing elements from an array without modifying the original array
The slice()
method can also be used to remove elements from an array without modifying the original array.
Assume we have an integer array ranging from 1 to 5
. You can use the following technique to remove the number 3 from the array without modifying the original array:
const numbers = [1, 2, 3, 4, 5];
const removedNumbers = numbers.slice(0, 2).concat(numbers.slice(3));
console.log(removedNumbers)
// Output: [1, 2, 4, 5]
In the preceding example, the first slice()
method will extract items from the index 0 to 2
. The next slice method will extract elements from index 3
the end of the array. The concat()
method is used to combine the results of both slice()
methods.
Difference between slice() and substring() method
The slice()
and JavaScript substring() methods both allow you to extract a portion of a string, but they have some differences:
Slice() | Substring() |
---|---|
The slice() JavaScript method allows you to extract a portion of a string or an array. | The substring() method only allows you to extract a portion of a string. |
The slice() string method also allows you to use negative index values. | The substring() does not support a negative index. |
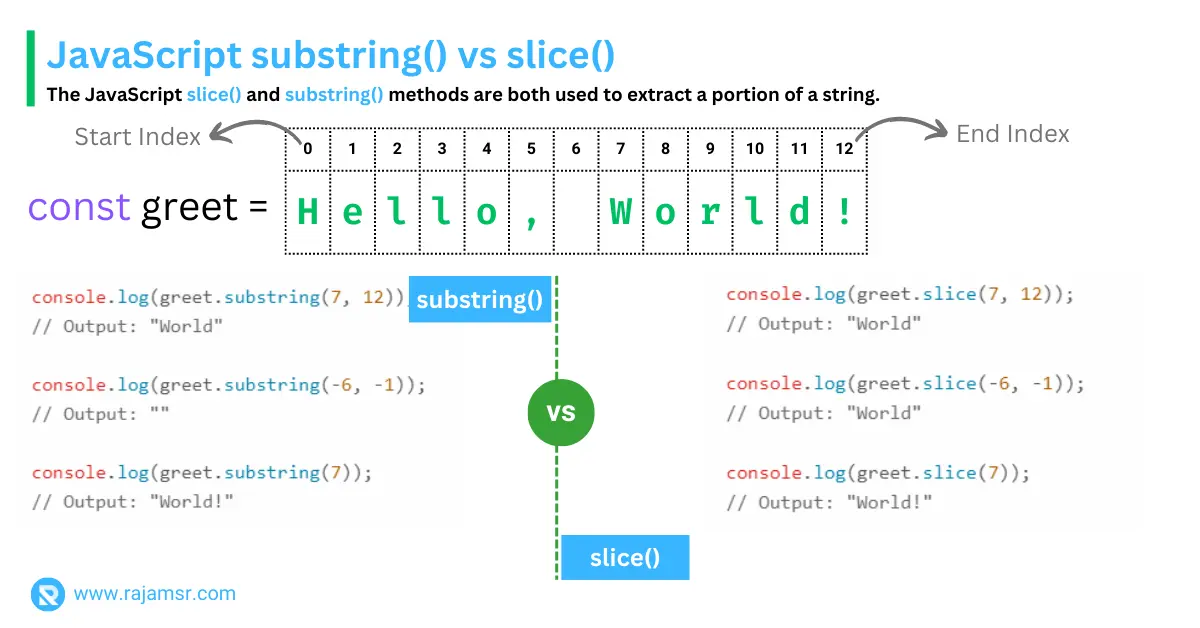
Conclusion
In conclusion, the JavaScript slice()
method provides an effective means of extracting a section of a string or array and producing a new string or array. Its versatility makes it a valuable function in many practical scenarios.
We covered three common usages of the slice()
in JavaScript, like extracting a portion of the string, copying an array, and removing array elements without modifying the original array.
This article explored the distinctions between the slice() and substring()
methods and highlighted three common mistakes to avoid when using the slice()
method in application code.