If you are a web developer, you probably use JavaScript arrays a lot. But do you know how to get the JavaScript array length
? You might think it’s as simple as using the length property, but there’s more to it than that. The length
property allows you to determine the number of elements in an array.
In this blog post, we’ll explore:
- JavaScript array length property
- Handling array length check
- Dealing with array length 0
- How to handle an array whose length is undefined
- The time complexity of array length calculation
- How to handle an array with less than 0
Let’s see each topic in detail.
What is a JavaScript array?
Before going into array length
, let’s see what a JavaScript array is.
A single variable in JavaScript can hold a collection of elements, which is known as array
. It can hold any data type and is defined using square brackets. The array is one of the non-primitive data types in JavaScript.
const jsArray = [1, "two", true, null, { 'language': 'JavaScript' }];
console.log(jsArray);
// Output: [1, "two", true, null,{'language':'JavaScript'}]
console.log(jsArray[1])
// Output: "two"
In this example, we’ve defined an array jsArray
that holds five elements of different data types.
You can access individual array elements using indexes, like jsArray[0]
for the first element, jsArray[1]
for the second, and so on. The array length count in JavaScript starts with 1
. However, the JavaScript index starts with 0
.
You can use the JavaScript indexOf() method to search an array.
Understanding JavaScript array length or size
Using the array length property
(not function
), we can set or get the number of elements in the array. You can access the length
property of an array using dot (.) notation, like this:
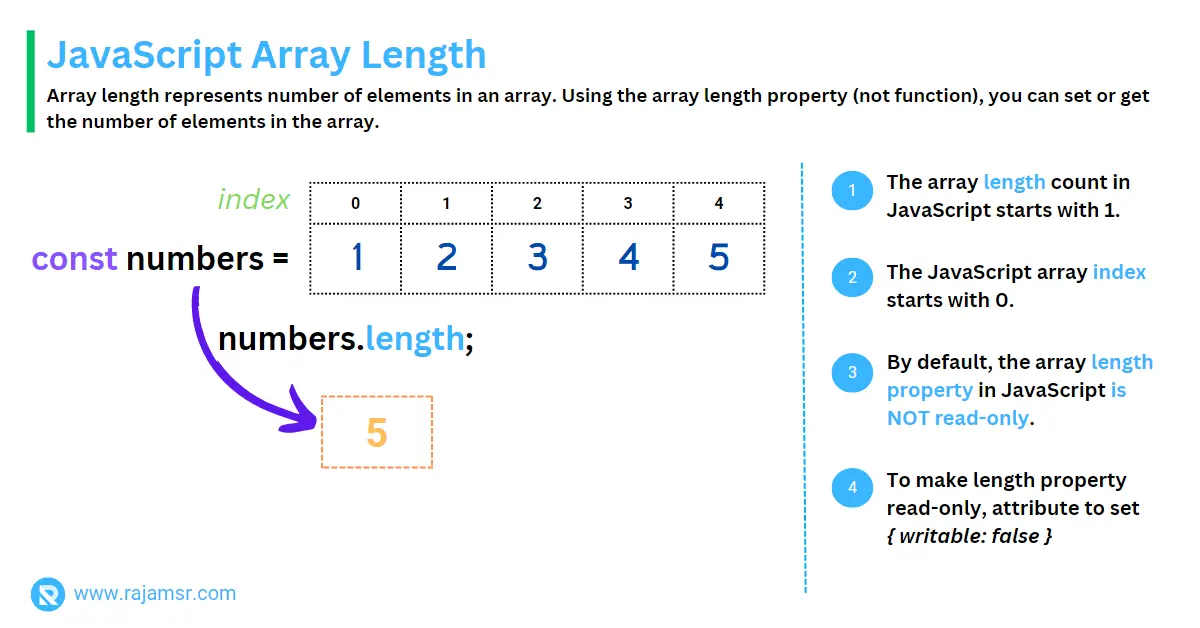
const numbers = [1, 2, 3, 4, 5];
console.log(numbers.length);
// Output: 5
In the above code example, the length
property returns the length of the array. Alternatively, you can calculate the length
by the array’s highest index plus 1.
Reduce the array length
If you assign a new value to the length
property, it will affect the number of elements in the array. Let’s see an example of how to set array length:
const numbers = [1, 2, 3, 4, 5];
numbers.length = 3;
console.log(numbers);
// Output: [1, 2, 3]
In the example above, we’ve tried to set the length
of the array to 3
. Setting the length
property to a value smaller than the current length truncates the array to that size. In this example, the actual length is 5
, but we are setting it to 3
. So the last two elements of the array have been removed. The value of the first three elements remains the same.
Increase the array length
When the length
is set to a bigger value than the current length, the array is extended by adding empty slots, not actual undefined
values
const numbers = [1, 2, 3, 4, 5];
numbers.length = 10;
console.log(numbers.length);
// Output: 10
console.log(`Array length: ${numbers.length}, values: ${numbers}`)
// Output: Array length: 10, values: 1,2,3,4,5,,,,,
In the above example, the actual array length is 5
. We are increasing to 10
, so the additional slots will be filled with empty ones. Finally, the result is printed to the console using the JavaScript string concatenation.
How to check array length in JavaScript?
Checking the length
of an array is an important operation when working with arrays. Using the length
property of the array is one of the most common approaches.
const numbers = [1, 2, 3, 4, 5];
if (numbers.length > 0) {
console.log(`Array has ${numbers.length} elements.`);
}
// Output: "Array has 5 elements."
Alternate approach to get array length using forEach() loop
The length
property will throw exceptions if it is accessed on a type other than a string or an array. So, it is usually preferable to check the data type if you are getting input from another method.
A safe way to check the length of an array is to use the Array.isArray()
method. It returns true
if the passed-in value is an array; otherwise, it returns false
.
const numbers = [1, 2, 3, 4, 5];
if (Array.isArray(numbers) && numbers.length > 0) {
console.log(`Array has ${numbers.length} elements.`);
}
If you don’t want to use an array length
property to check the length, you can use forEach()
or a for loop
to iterate through the array elements to find the length of an array. Use this approach only if you can’t use the built-in length property.
In the following example, the forEach()
loop is used to find the array length:
const months = ['Jan','Feb','Mar', 'Apr', 'May'];
let length = 0;
months.forEach(e => length++)
console.log(length);
// Output: 5
Make length property read-only
By default, the array length property in JavaScript is not read-only
. Allowing to change the length property leads to unexpected results sometimes. So, how do you prevent it? Simple. You can set that property’s writable
attribute to false
, as shown in the following example:
const numbers = [1, 2, 3, 4, 5];
Object.defineProperty(numbers, "length", { writable: false });
numbers.length = 3
console.log(numbers.length)
// Output: 5
How to use length property in real-time use case
In real-time use cases, most of the time, length
property is used before doing the actual operation. For example, you have an input email address
and you want to extract the domain name
from it.
The @ delimiter
can be used in combination with the JavaScript String split()
method. The output will be returned as an array. You must verify that the array length is 2 before extracting the domain. If the given email address doesn’t have an @ symbol
and we access the array element without validating its length, it will return undefined
.
let email = 'john.doe@gmail.com'
let tokens = email.split('@')
if (tokens.length === 2) {
console.log(`Email domain is ${tokens[1]}`)
}
else {
console.log('Invalid email format.')
}
You can split an array of elements into chunks, in that case, you can use the JavaScript array method split() method.
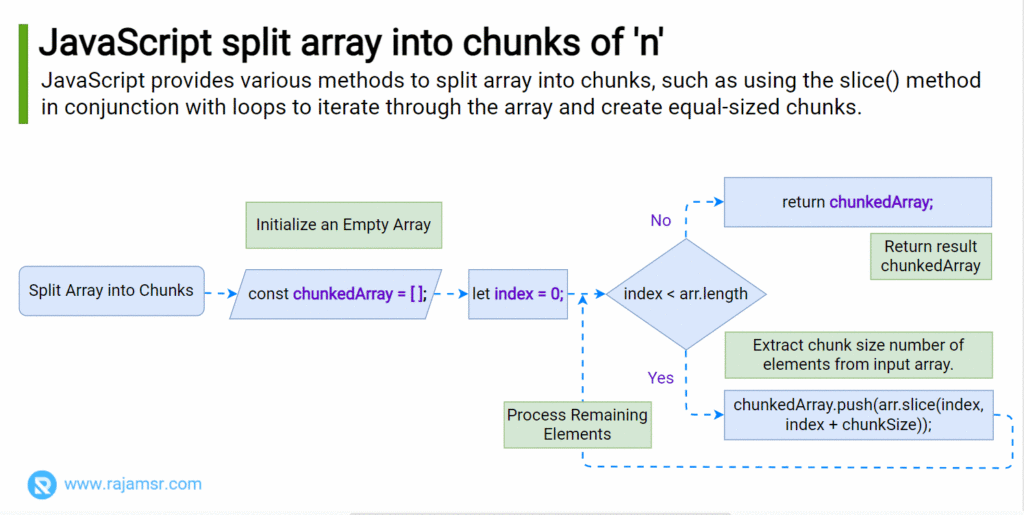
Dealing with array length 0
An array with the length 0 is an empty array. There are several reasons why an array might be empty, such as when it is initialized or when all of its elements have been removed. You can check if an array object is empty by comparing its length
property to 0
.
const emptyArray = [];
if (emptyArray.length === 0) {
console.log("The array is empty.");
}
Setting the length property to 0 is one of the ways to clear an array in JavaScript.
Empty arrays can be useful in certain situations, such as when you want to create an array that will be populated later.
const newArray = new Array(5).fill(null);
console.log(newArray);
// Output: [null, null, null, null, null]
In the example above, we’ve created an array with a length of 10
, but we haven’t initialized it with any values yet.
Array length undefined
When a JavaScript array length
is undefined
, it means that the array is empty
or uninitialized
. To set the length of an array, you can assign a new value to its length property:
const numbers = [1, 2, 3];
console.log(numbers.length);
// Output: 3
numbers.length = 5;
console.log(numbers);
// Output: [1, 2, 3, undefined, undefined]
In the example above, we’ve set the length
of the array to 5
. Since the array only had three elements
, the last two elements were undefined
.
The time complexity of array length calculation
The time complexity of accessing an array’s length property is O(1)
, which means that it takes constant time regardless of the size of the array. This is because the length property is a built-in property of the array object and is always stored as a single value.
To confirm, I did performance benchmark testing using jsPerf. The results are interesting!
I made two number arrays, one with 1000 elements
and the other with 10000
. I was able to perform 165,658
array length calculation operations per second on the 10000-element
array.
However, I was only able to perform 159,668
array length calculations per second on the 1000-element array, which is 4% slower than huge array length calculations.
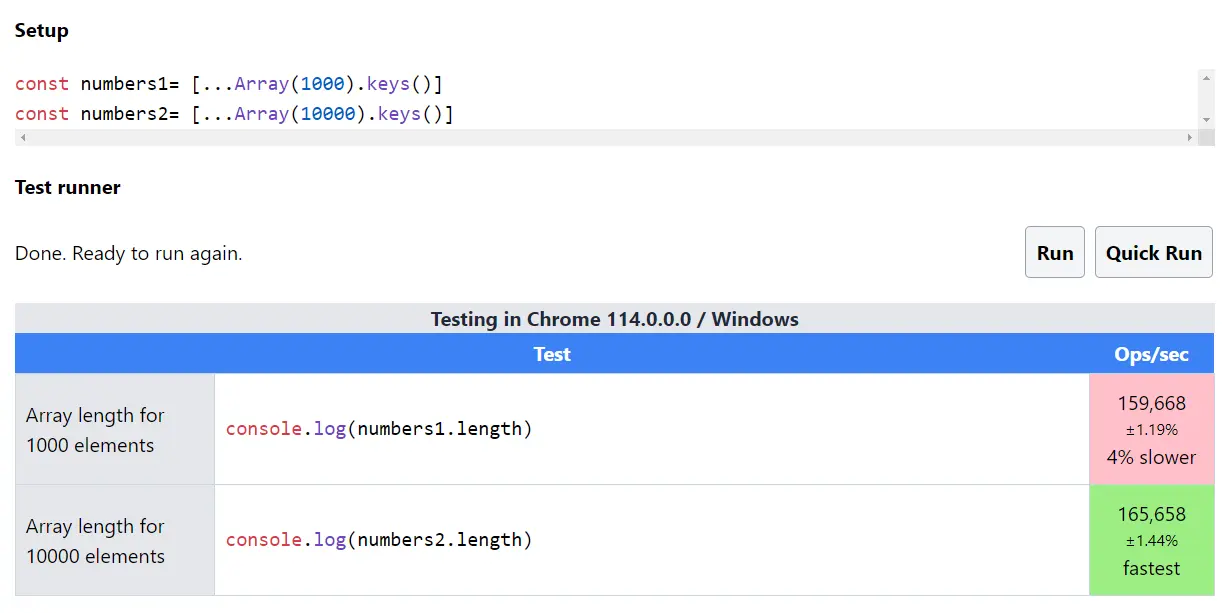
Array length is less than 0
An array’s length
property can never be less than 0
. If you try to set the length
property of an array to a negative value-1
, it will throw an "Invalid array length"
error:
const myArray = [1, 2, 3];
console.log(myArray.length);
// Output: 3
myArray.length = -1;
// RangeError: Invalid array length
In the example above, we tried to set the length
of the array to -1
, but it displayed an error as expected.
Array length is 1 but empty
An array with length 1 but no elements is a special case in JavaScript. In this case, the array is considered to be "sparse"
, which means that it has a length of 1 but no elements.
The length property of an array typically returns the exact number of elements in the array, but with sparse arrays, the value of the length is higher than the number of items in the length property.
You can create a sparse array like this:
const sparseArray = new Array(1);
console.log(sparseArray);
// Output: []
console.log(sparseArray.length);
// Output: 1
sparse array
with a length of 1. Since the array has no elements, its only element is undefined
.Conclusion
The length
property is an important feature of JavaScript arrays that allows us to determine the number of elements in an array. In this blog post, we’ve covered everything you need to know about the JavaScript array length
property, from how to access it using the length property.
If you can’t use the built-in length property, using a loop
, you can calculate the array length. Now you know how to handle special cases like empty arrays
, undefined lengths
, and sparse arrays
.
Understanding the array length property
is essential for working with arrays in JavaScript and will help you write more efficient and effective code.