If you are a JavaScript developer, you might have heard of the term non primitive data types in JavaScript. But do you know what they are, how they differ from primitive data types, and why they are important for your code?
Non primitive data types are complex data types that can store multiple values or objects in a single variable. Unlike primitive data types, such as numbers, strings, booleans, null, and undefined, non primitive data types are mutable, meaning they can be modified after they are created.
Some examples of non primitive data types in JavaScript are arrays, objects, functions, and dates. These data types allow you to create dynamic and flexible data structures that can handle various scenarios and tasks.
In this blog post, we will explore:
- What is non-primitive data type?
- Different non primitive data types in JavaScript
- Features and benefits of non primitive types in JavaScript
- Difference between primitive and non-primitive data types
By the end of this post, you will have a better understanding of non primitive data types in JavaScript. Let’s dive in.
What is non primitive data type in JavaScript?
Data structures that allow you to store and manage collections of data are non-primitive data types
. We can also call them reference data types
because they hold a reference to the actual data, not the data itself.
Understanding non-primitive data types is important because they provide a way to store and manage data in a structured manner, which can make your code more organized and easier to maintain.In addition to this, the type of data you are creating, like classes or objects, also falls under the non-primitive data type.
Different non primitive data types in JavaScript
There are several non-primitive data types in JavaScript, including:
- Arrays
- Objects
- Functions
- Maps
- Sets
- WeakMaps and WeakSets
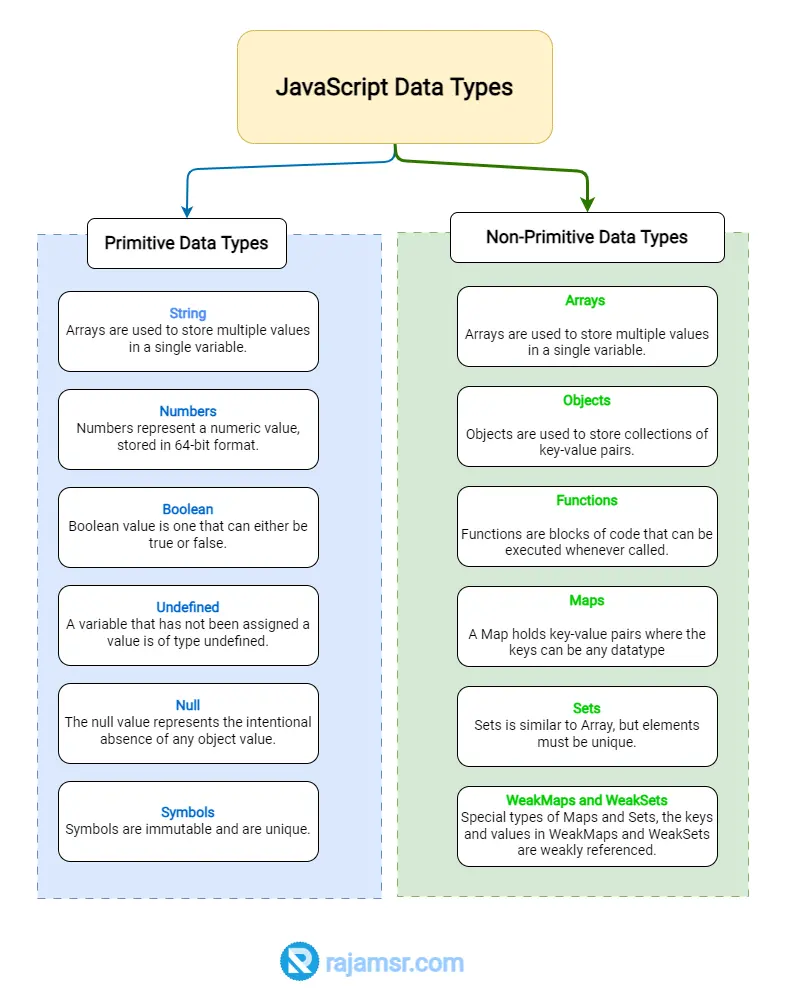
1. Arrays
Arrays
are one of the most commonly used non primitive data types in JavaScript. They are used to store collections of values and can be accessed using an index.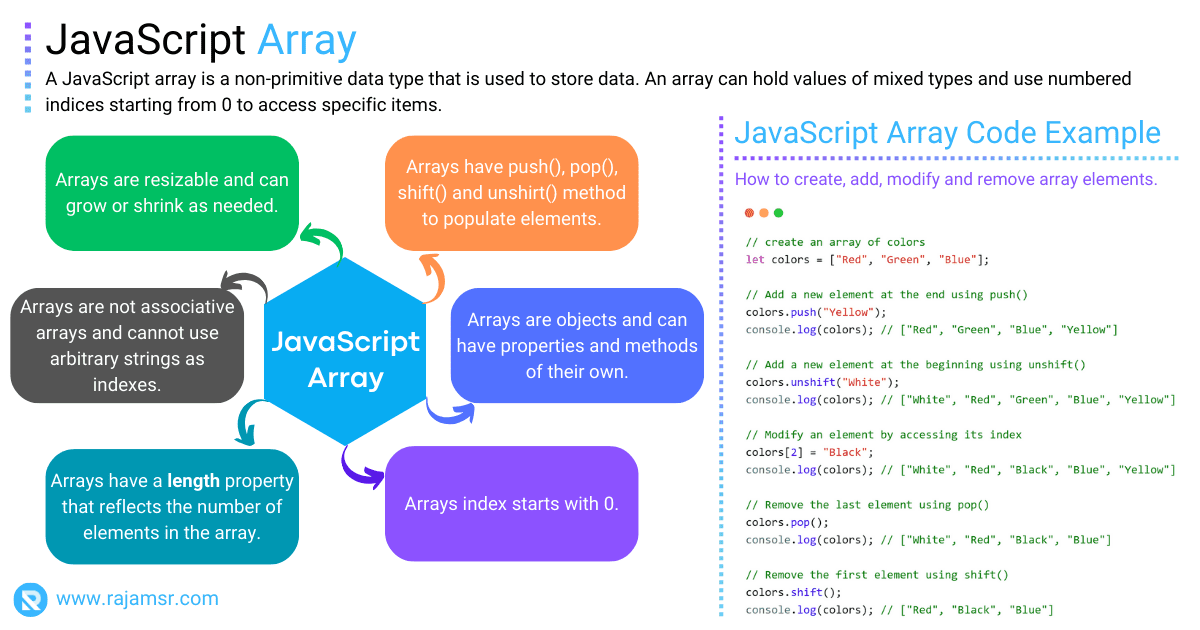
Creating arrays
square brackets
. For example:let numbers = [1, 2, 3, 4, 5];
Using the JavaScript array length property, you can determine the number of elements in an array.
Accessing elements in an array
Access elements in an array using their index. The JavaScript index starts at 0
. For example, an index 0
returns the first element from an array.
let numbers = [1, 2, 3, 4, 5];
let firstNumber = numbers[0];
// Output: 1
Modifying elements in an array
array
by assigning a new value to the element at the desired index. For example:let numbers = [1, 2, 3, 4, 5];
numbers[0] = 10;
console.log(numbers)
// Output: [10, 2, 3, 4, 5]
Array methods
There are several built-in JavaScript array methods that you can use to manipulate arrays. Some common array methods include the following:
Method | Description |
---|---|
push() | JavaScript push() method adds an element to the end of an array. |
pop() | Removes the last element from an array. |
shift() | JavaScript shift() method removes the first element from an array. |
unshift() | The JavaScript unshift() adds an element to the beginning of an array. |
slice() | JavaScript slice() method returns the selected elements from an array, as a new array. |
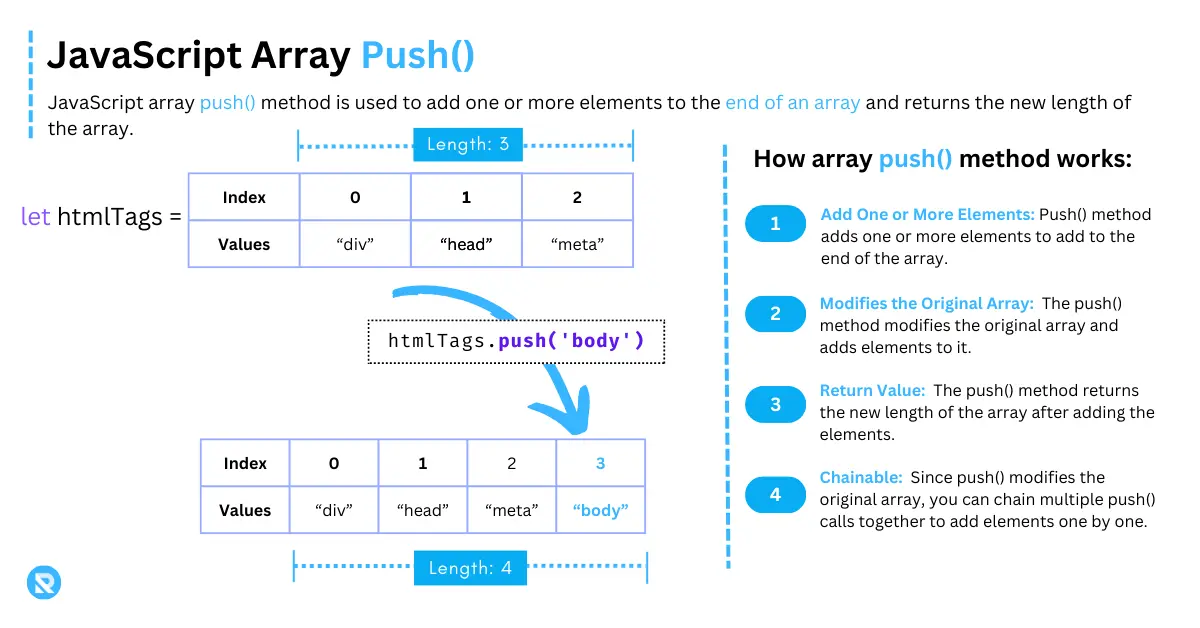
2. Objects
Objects
are another commonly used non-primitive data type in JavaScript. They are used to store collections of key-value pairs, or JavaScript dictionaries, where the key is a string and the value can be of any data type.
Built-in static methods such as Object.keys()
, Object.values()
, and Object.entries()
help you to access object keys and values in the Objects
.
Method | Description |
---|---|
Object.keys() | JavaScript Object.keys() method returns object key names as an array. |
Object.values() | Object.values() returns object key values as an array. |
Object.entries() | JavaScript Object.entries() returns Keys and Values as an Array. It returns the key-value pair as an array of arrays. |
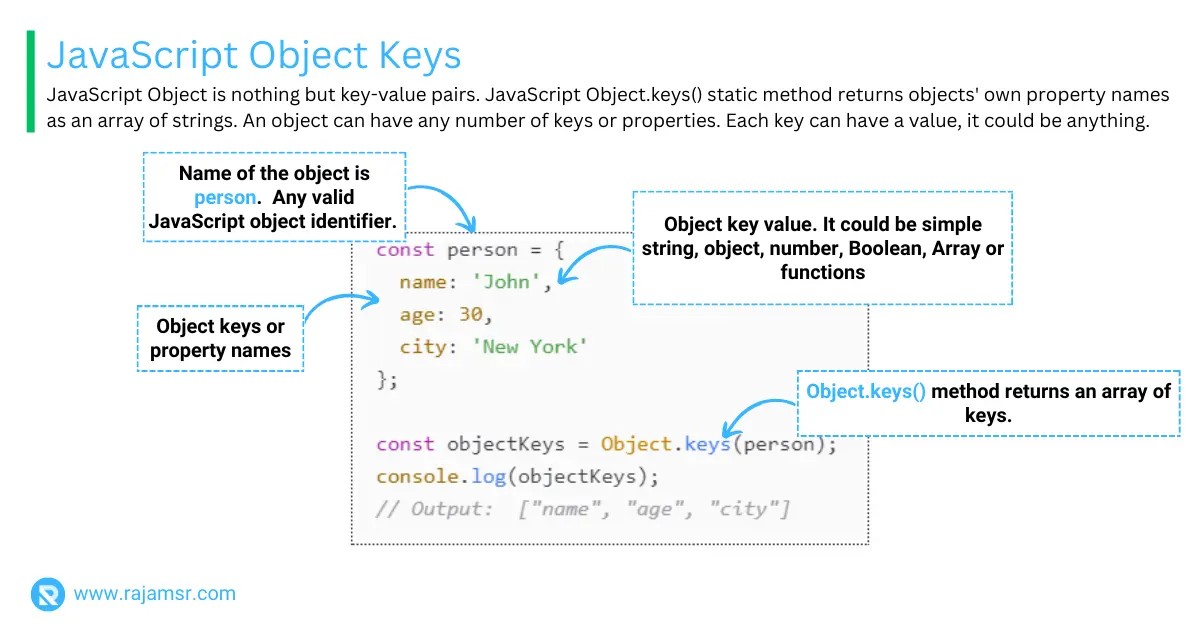
Creating Objects
Create an object in JavaScript using curly braces. For example, the following code creates the person
object with name
, age
and job
properties:
let person = {
name: "John Doe",
age: 25,
job: "Full Stack Developer"
};
Accessing properties in an Object
dot notation
or square bracket notation
. For example:let person = {
name: "John Doe",
age: 25,
job: "Full Stack Developer"
};
let name = person.name;
// Output: "John Doe"
let age = person["age"];
// Output: 25
Modifying properties in an Object
Modify properties in an object by assigning a new value to the property. For example:
let person = {
name: "John Doe",
age: 25,
job: "Full Stack Developer"
};
person.age = 30;
It is possible to conditionally add keys to an object in JavaScript.
Object methods
Add methods to an object by adding a function to a property. For example:
let person = {
name: "John Doe",
age: 30,
job: "Full Stack Developer",
greet: function() {
console.log(`Hello, This is ${this.name}.`);
}
};
person.greet();
// Output: "Hello, This is John Doe."
3. Functions
A function is a code block that runs when you invoke it
. It performs a specific task and can take parameters and return a value. Let’s see what we can do with functions.
Creating functions
Using the function
keyword, you create a function in JavaScript. For example:
function greet(name) {
console.log(`Hello, ${name}.`);
}
Invoking functions
To invoke a function, you can call it using its name. For example:
function greet(name) {
console.log(`Hello, ${name}.`);
}
greet("World");
// Output: "Hello, World."
Functions as Objects
In JavaScript, functions are not only actions but also values that can be assigned to variables or passed as arguments. Like any other value in JavaScript, functions have properties and methods
that describe their characteristics and behavior. For example:
function greet(name) {
console.log(`Hello, ${name}.`);
}
greet.role = "Full Stack Developer";
console.log(greet.role);
// "Full Stack Developer"
4. Maps
Maps
are a newer data structure in JavaScript that was introduced in ECMAScript 6. They are similar to objects but have a few key differences. The main difference is that the keys in a Map
can be any data type, not just strings.
Creating Maps
Create a Map
in JavaScript using the new Map()
constructor. For example:
let map = new Map();
map.set("name", "John Doe");
map.set("age", 25);
map.set("job", "Full Stack Developer");
Accessing elements in a Map
Access elements from Map
using the get()
method. For example:
let map = new Map();
map.set("name", "John Doe");
map.set("age", 25);
map.set("job", "Full Stack Developer");
console.log(map.get('name'))
// Output:"John Doe"
Modifying elements in a Map
Modify elements in a Map
by using the set()
method to assign a new value to a key. For example:
let map = new Map();
map.set("name", "John Doe");
map.set("age", 25);
map.set("job", "Full Stack Developer");
// Modify the value of role
map.set('job', 'FSE')
Iterating over a Map
Iterate over a Map
using for...of
loops or using the forEach()
method. For example:
let map = new Map();
map.set("name", "John Doe");
map.set("age", 25);
map.set("job", "Full Stack Developer");
for (let [key, value] of map) {
console.log(`${key}: ${value}`);
}
// Output:
"name: John Doe"
"age: 25"
"job: Full Stack Developer"
5. Sets
Sets
are one of the non-primitive data types in JavaScript. Sets
are a newer data structure in JavaScript that was introduced in ECMAScript 6
. They are similar to arrays but have a few key differences.
The main difference is that the elements in a Set must be unique.
Creating sets
Create a Set
in JavaScript using the new Set()
constructor. For example:
let months = new Set();
months.add("Jan");
months.add("Feb");
Adding elements to a Set
Add elements to a Set
using the add()
method. For example:
let months = new Set();
// Add Elements to Set using .add() method
months.add("Jan");
months.add("Feb");
Removing elements from a Set
Set
using the delete()
method.- Values are
case-sensitive
. Before adding a string value to a Set, you can convert it to JavaScript lowercase usingtoLowerCase()
or to JavaScript uppercase using thetoUpperCase()
method. - It will not throw any exception if the value is not on the list.
let months = new Set();
months.add("Jan");
months.add("Feb");
months.add("Mar");
months.delete("Mar");
console.log(months)
// Output
0:"Jan"
1:"Feb"
Iterating over a Set
Iterate over a Set
using for...of
loops or using the forEach()
method. For example:
let months = new Set();
months.add("Jan");
months.add("Feb");
months.add("Mar");
for (let month of months) {
console.log(month);
}
// Output
"Jan"
"Feb"
"Mar"
Converting Sets to Arrays
Set
to an array using the Array.from()
method. For example:let months = new Set();
months.add("Jan");
months.add("Feb");
months.add("Mar");
let names = Array.from(months);
console.log(names)
// Output: ["Jan", "Feb", "Mar"]
Checking if an element exists in a Set
Set
using the has()
method. Remember, value is case-sensitive. For example:let months = new Set();
months.add("Jan");
months.add("Feb");
months.add("Mar");
let monthExists1 = months.has("Jan"); // true
let monthExists2 = months.has("jan"); // false
Using Sets to remove duplicates from Arrays
Sets
is to remove duplicates from arrays. For example:let array = ["Jan", "Feb", "Mar", "Jan"];
let set = new Set(array);
let uniqueArray = Array.from(set);
console.log(uniqueArray)
// Output: ["Jan", "Feb", "Mar"]
6. WeakMaps and WeakSets
WeakMaps
and WeakSets are special types of Maps
and Sets
, respectively, that have a few key differences. The main difference is that the keys and elements in WeakMaps
and WeakSets
are weakly referenced. This means that if the only reference to an object is in a WeakMap
or WeakSet
, the object can be garbage collected, even if it hasn’t been explicitly removed from the WeakMap
or WeakSet
.
Creating WeakMaps and WeakSets
Create a WeakMap
or WeakSet
in JavaScript using the new WeakMap()
and new WeakSet()
constructors, respectively. For example:
let weakMap = new WeakMap();
weakMap.set({}, "value");
let weakSet = new WeakSet();
weakSet.add({});
Creating WeakMaps and WeakSets
WeakMaps
using the set()
method and elements to WeakSets
using the add()
method. For example:let weakMap = new WeakMap();
weakMap.set({}, "value");
let weakSet = new WeakSet();
weakSet.add({});
Removing elements from WeakMaps and WeakSets
Unlike regular Maps
and Sets
, WeakMaps
and WeakSets
do not provide any methods to explicitly remove elements.
Iterating over WeakMaps and WeakSets
WeakMaps
and WeakSets
do not provide any methods for iterating over their keys and elements. This is because the keys and elements in WeakMaps
and WeakSets
are weakly referenced and can be garbage collected at any time.
Difference between primitive and non-primitive data types
Basic data types that are immutable
, meaning they cannot be modified, are primitive data types in JavaScript. They are stored in memory and accessed directly.
Complex data types that are mutable, meaning their values can be modified, are non-primitive data types, on the other hand.
Here are the fundamental differences between primitives
and non-primitive data types
in JavaScript:
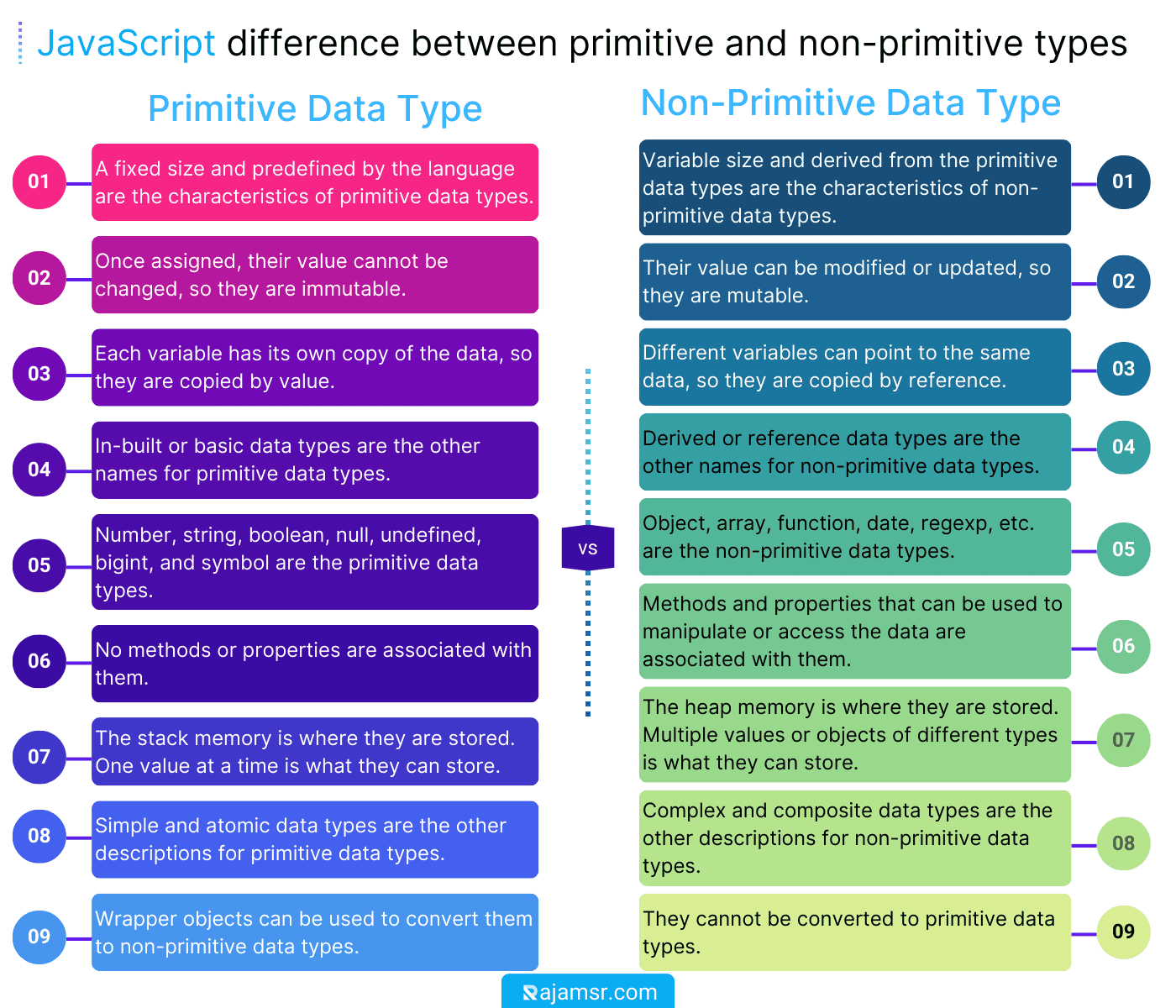
Conclusion
In this article, we have covered the non primitive data types
in JavaScript, including Arrays
, Maps
, Sets
, WeakMaps()
, and WeakSets()
. We discussed their definitions, applications, and distinctions.
We use arrays to store data in ordered collections, and we use Maps
and Sets
to store key-value pairs and unique values, respectively.
Weak references to keys and elements allow WeakMaps
and WeakSets
to be garbage collected when they become unnecessary, unlike Maps
and Sets
.
It’s important to understand the differences between these non-primitive data types and choose the right one for your use case to ensure efficient and effective data management in your JavaScript code.
I hope this article has provided you with a good understanding of non-primitive data types in JavaScript.